Introduction
To improve performance and provide a smoother process, our API supports asynchronous workflows and requests. Our asynchronous functionality can be divided into:
- Asynchronous historical data workflow (for single links)
Removes the need for POST calls after link creation to retrieve core information about the link. - Asynchronous historical data workflow (for recurrent links)
Removes the need for POST calls after link creation to retrieve core information about the link and enables you to receive updates for any changes in the information. - Asynchronous real-time POST calls (both single and recurrent links)
Eliminate the risk of timeouts and improve your data flow.
Asynchronous historical data workflow (single links)
Whether you create a single link via our API or use the widget to create your links, you can choose to asynchronously receive the historical information for your user by adding the fetch_resources
parameter to your request:
curl --request POST \
--url https://sandbox.belvo.com/api/token/ \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '{see request body below}'
{
"id": "YOUR_SECRET_ID",
"password": "YOUR_SECRET_PASSWORD",
"external_id": "asynchronous-historical-request",
"scopes": "read_institutions,write_links",
"fetch_historical": true,
"fetch_resources": [
"FINANCIAL_STATEMENTS",
"INVOICES",
"TAX_RETURNS",
"TAX_STATUS",
"TAX_COMPLIANCE_STATUS",
"TAX_RETENTIONS"
]
}
Once the link is created, Belvo will retrieve all the data available for the resources you’ve specified and notify you via webhook once the information is ready for you to retrieve:
For more details regarding which resources you can send through, see our Register a new link request API reference.
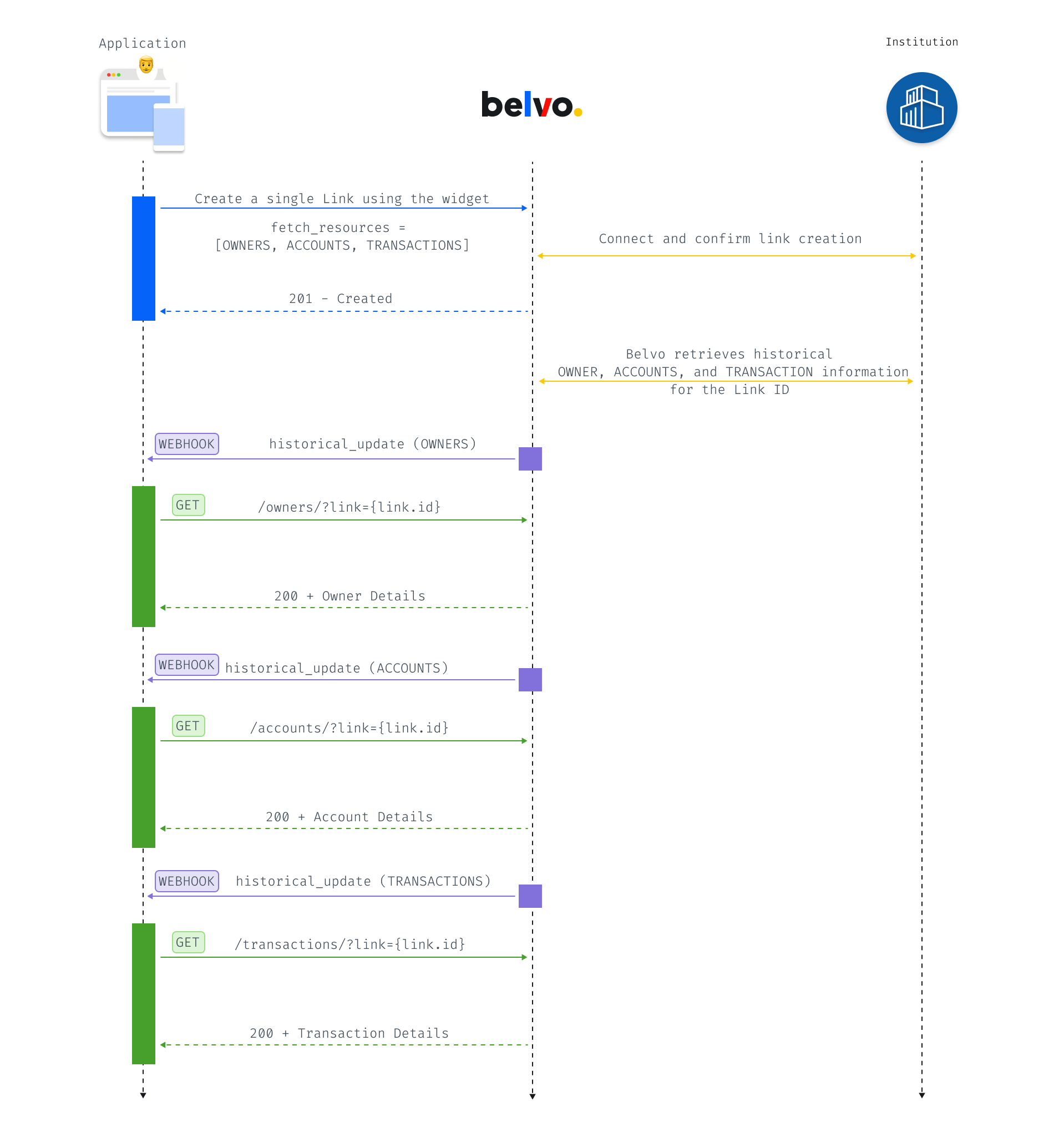
- Register a link using the Connect Widget
When you generate your access_token, make sure to pass thefetch_resources
parameter. Once your user connects to their account using our Connect Widget, Belvo will respond with the linkid
and start to asynchronously load the resources you listed infetch_resources
. - Wait for webhooks
As soon as we retrieve data for each resource you listed, you'll receive ahistorical_update
webhook for that resource. For each webhook you receive, you need to make a GET List request to Belvo to get the data.
In the code block below, you can see an example of the webhook you will receive and the request you need to send to retrieve the data.{ "webhook_id": "aadf41a1fc8e4f79a49f7f04027ac999", "webhook_type": "TRANSACTIONS", "webhook_code": "historical_update", "link_id": "16f68516-bcbc-4cf7-b815-c500d4204e28", // The link that the data belongs to "request_id": "4363b08b-51eb-4350-9c74-5df5ac92a7f6", "external_id": "optional_parameter_you_can_provide", "data": { "total_transactions": 19, // Total number of transactions found "total_inflow_transactions": 10, // Total number of inflow transactions "total_outflow_transactions": 9, // Total number of outflow transactions "first_transaction_date": "2017-01-03", // First transaction date "last_transaction_date": "2020-03-25" // Last transaction date } }
curl --request GET \ --url 'https://sandbox.belvo.com/api/transactions/?link=16f68516-bcbc-4cf7-b815-c500d4204e28' \ --header 'accept: application/json'
Asynchronous historical data workflow (recurrent links)
Whether you create a recurrent link via our API or use the widget to create your links, you will asynchronously receive the historical information for your user, along with regular updates to any changes to the user's information.
Once the link is created, Belvo will retrieve all the data available for the resources you’ve specified and notify you via webhook once the information is ready for you to retrieve:
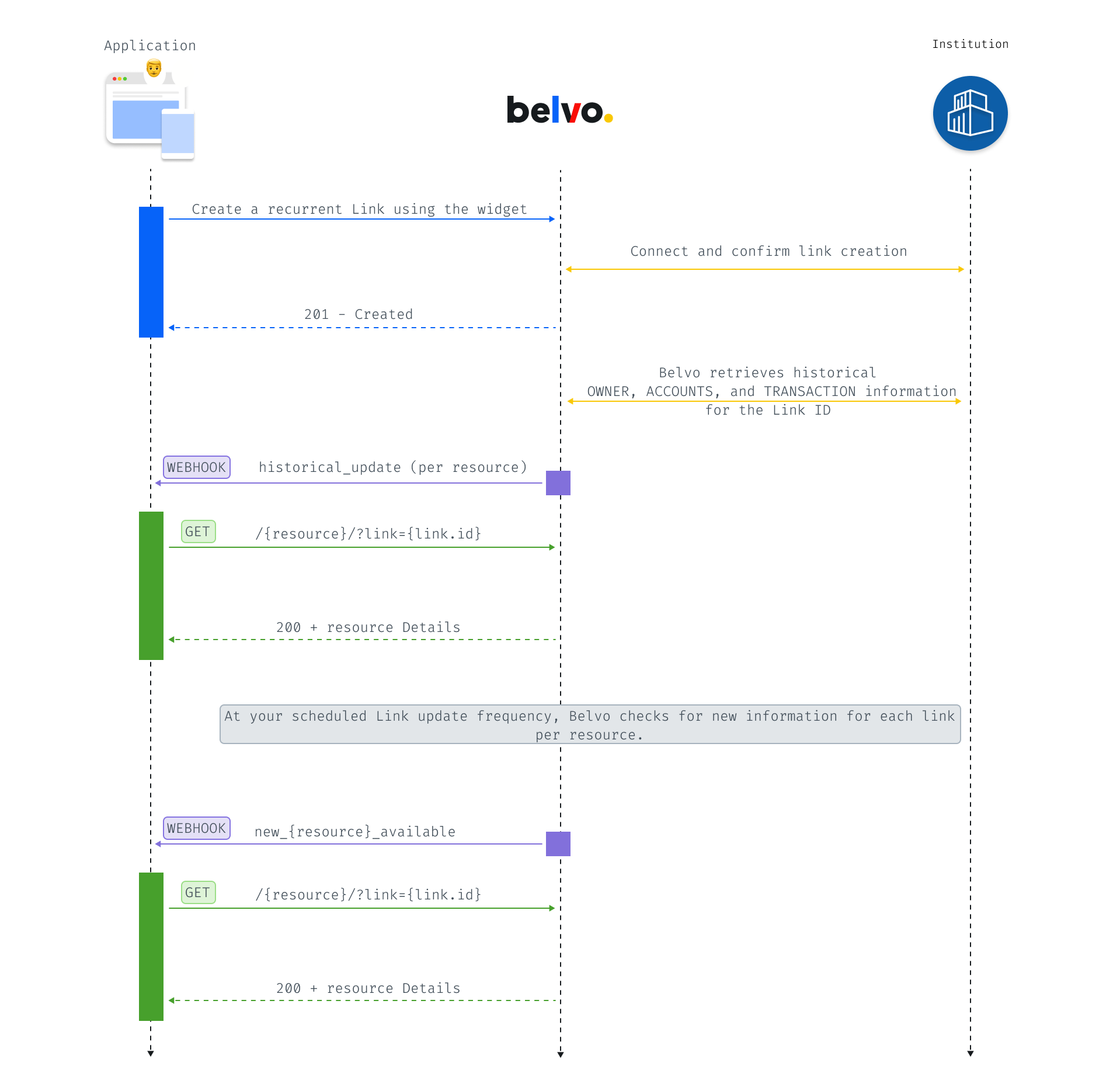
- Register a link using the Connect Widget
Once your user connects to their account using our Connect Widget, Belvo will respond with the linkid
and start to asynchronously load the core resources for the link type. - Wait for webhooks (historical)
As soon as we retrieve data for each resource, you'll receive ahistorical_update
webhook for that resource. For each webhook you receive, you need to make a GET List request to Belvo to get the data.
In the code block below, you can see an example of the webhook you will receive and the request you need to send to retrieve the data. - Wait for webhooks (new resource available)
Depending on your update frequency, we'll send you anew_{resource}_updated
webhook, indicating that we have retrieved new data for the link.
Asynchronous real-time POST calls (both single and recurrent links)
By making your individual POST calls asynchronous, you eliminate the risk of receiving timeout errors and can design your information aggregation in a more expected manner.
📘 At the moment, our asynchronous real-time POST call applies to the following requests:
- POST Retrieve Transactions
- POST Retrieve Invoices
- POST Retrieve Employments in Brazil
- POST Retrieve Employment Records in Mexico
- POST Retrieve Financial Statements in Mexico
We are continuously rolling out this feature to our remaining POST methods.
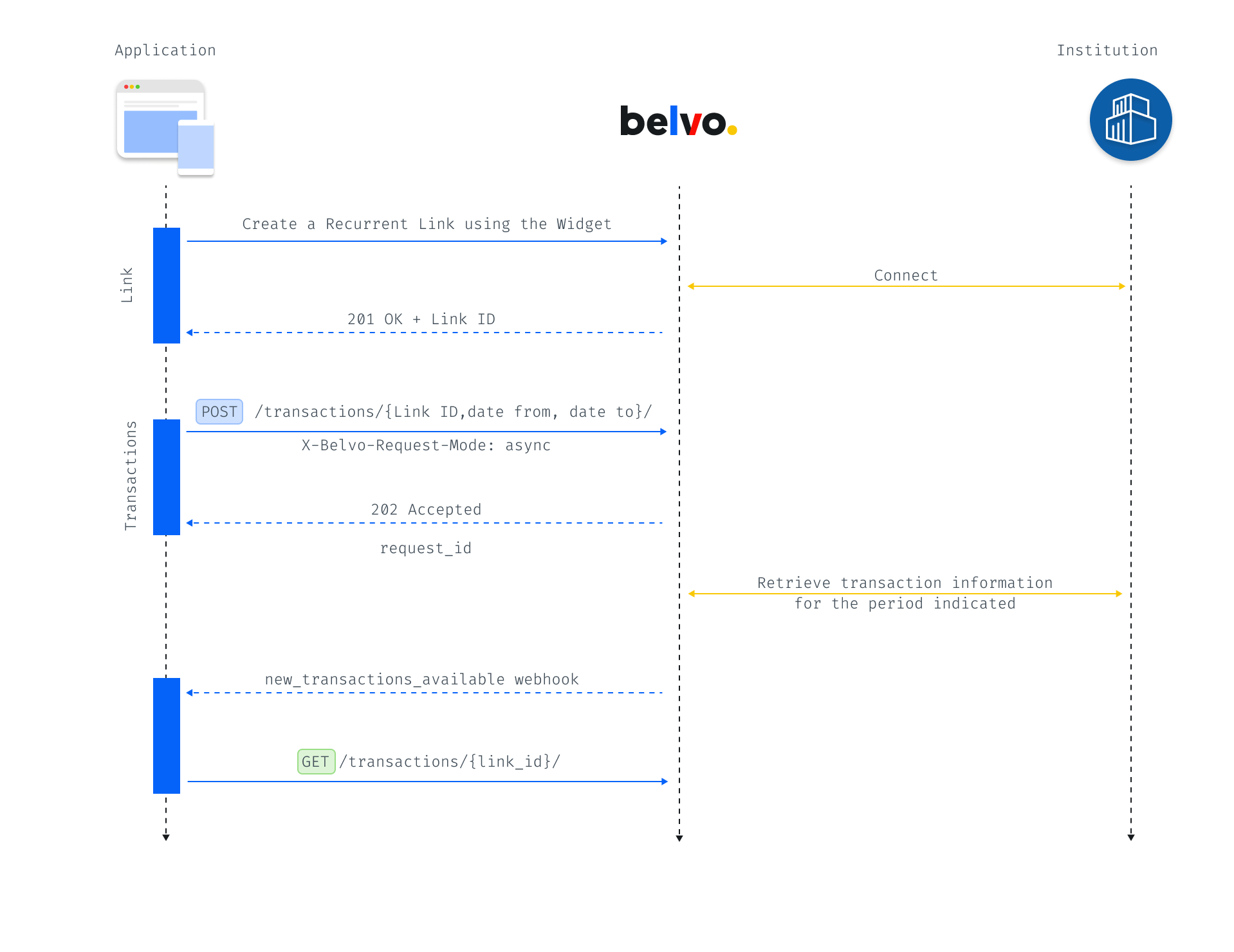
- Register a link using the Connect Widget
Your user connects to their account using our Connect Widget. After they've successfully connected, you'll receive a Link ID that you'll need to use in order to make further requests about the user. - Send a POST request with new
async
header
Make a POST call usingX-Belvo-Request-Mode
set toasync
.
You'll receive a202 - Accepted
response with arequest_id
in the body. We recommend you store thisrequest_id
so later when you receive a webhook you'll know to which request the webhook is in response to. - Wait for webhook
As soon as you make your POST request, Belvo will begin retrieving data about the user. We send a webhook once all the requested information has been retrieved. When you receive the webhook, you can make a GET request to the appropriate endpoint to retrieve the information.
curl --location --request POST 'https://sandbox.belvo.com/api/transactions/' \
--header 'X-Belvo-Request-Mode: async' \
--header 'Authorization: Basic ' \
--header 'Content-Type: application/json' \
--data-raw '{
"link": "bcca0da9-a4c6-4830-9676-1d54682851d9",
"date_from": "2022-12-17",
"date_to": "2023-01-16"
}
curl --location --request POST 'https://sandbox.belvo.com/api/invoices/' \
--header 'X-Belvo-Request-Mode: async' \
--header 'Authorization: Basic ' \
--header 'Content-Type: application/json' \
--data-raw '{
"link": "bcca0da9-a4c6-4830-9676-1d54682851d9",
"date_from": "2022-12-17",
"date_to": "2023-01-16",
"type": "OUTFLOW"
}
{
"request_id": "3f58b8a6f025402f8de4a4cc55d38705"
}
Manually triggering a historical update
You can also manually trigger an asynchronous historical update for a link's data by using our Trigger a historical update for a link method.
The flow is very similar to when you first create a link:
- You make a
POST
request to the endpoint. - Belvo returns a
202 Accepted
response with arequest_id
. - When the data is ready, Belvo sends a
historical_update
webhook. - You can then make a
GET
request to retrieve the updated data.
To prevent duplicate requests, the Refresh historical data for a link endpoint has a 10-minute cooldown period for each link. If you make a request for the same link within this period, you will receive a synchronous 409 Conflict
error.
Best Practices
Do I need to send up a webhook URL even for single links?**
Yes, you will need to have webhooks set up to make use of our asynchronous functionality.
Do I need to response to the webhook when I receive it?
Yes, once you receive a webhook send a 202 - Accepted webhook
to Belvo within five seconds to confirm that you have received the webhook. Otherwise, our API will retry the request.
If you see any issues with the JSON, please respond to Belvo with a 400 Bad Request
, including the request ID.
Can I use Asynchronous POST requests for any type of link?
Yes! Whether the link is single or recurrent, whenever you make a POST call you can use the async
header.