Android (Kotlin)
Check out some examples of how to set up deeplinks and listen for webview redirects in Android 🤖.
Prerequisites
In order to be able to use the hosted widget in your Android app, make sure that you:
- Can create access_tokens in your server-side.
- Know how to implement webviews for your platform. For more information, see the webview articles from Google.
Set up deeplinks in your Android application
In your AndroidManifest.xml
file, add the following code:
<manifest ...>
<!-- ... -->
<application ...>
<activity ...>
<!-- ... -->
<intent-filter> <!-- You need to add this entire XML object. -->
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accepts URIs that begin with your-url-here:// -->
<data
android:scheme="your-url-here"
android:host="" />
</intent-filter>
</activity>
</application>
</manifest>
Handle events in your webview
In the code sample below you can see an example of how to listen for and handle events within your webview.
val belvoWebView: WebView = findViewById(R.id. WEBVIEW_ID_ASSIGNED_IN_CONTENT )
belvoWebView.loadUrl("https://widget.belvo.io/?access_token={access_token_from_backend}")
belvoWebView.settings.javaScriptEnabled = true
belvoWebView.settings.allowContentAccess = true
belvoWebView.settings.setDomStorageEnabled(true)
belvoWebView.settings.useWideViewPort = true
belvoWebView.webViewClient = object : WebViewClient () {
override fun shouldOverrideUrlLoading(view: WebView?, url: String): Boolean {
view?.loadUrl(url)
if (url.startsWith("https")) {
return true
}
else {
belvoWebView.stopLoading()
val uri: Uri = Uri.parse(url)
val host: String? = uri.host
if (host == "success") {
val link : String? = uri.getQueryParameter("link")
val institution : String? = uri.getQueryParameter("institution")
// Do something with the link and institution.
}
else if (host == "exit") {
// If the redirect starts with "exit",
// Do something with the data.
}
else {
// If the redirect starts with "error",
// Do something with the data.
}
belvoWebView.goBack()
}
return false
}
}
belvoWebView.addJavascriptInterface(BelvoWidget(), "your-url-here") // Used to listen for additional events
Please note: You might receive a warning due to thebelvoWebView.settings.javaScriptEnabled = true
line (as in the image below). Belvo's Widget for Webviews fulfills all kinds of XSS mitigations, so we recommend suppressing this warning with the annotation suggested by Android Studio.
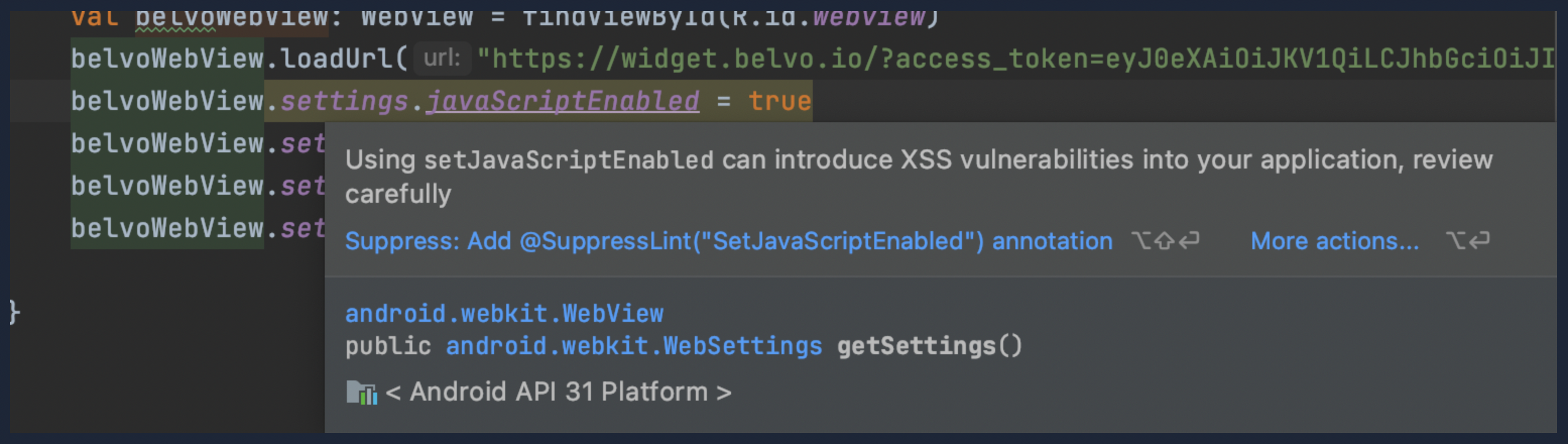
Possible warning message
Listening to additional events
Our hosted widget also sends additional data regarding events the user encounters throughout the widget. For example, when the user goes from the institution selection screen to the credentials login screen, our widget will send an event.
The events are sent through as a JSON string payloads with the following schema:
"{
"eventName": "PAGE_LOAD",
"metadata":{
"page": "/institutions", // Page that the user is directed to
"from": "/consent", // Page where the user was previously
"institution_name": "", // Note: This field only appears AFTER they've selected an institution
}
}"
"{
"eventName": "ERROR",
"request_id": "UUID",
"meta_data": {
"error_code": "login_error",
"error_message": "Invalid credentials provided to login to the institution",
"institution_name": "bancoazteca_mx_retail",
"timestamp": "2020-04-27T19:09:23.918Z"
}
}"
To listen to these events:
- Add the following class to your application.
class BelvoWidget() {
@JavascriptInterface
fun sendMessage(data:String) {
println( "Event data: $data" )
}
}
- Add the following line of code to the end of your logic for handling deep links in your webview:
belvoWebView.addJavascriptInterface(BelvoWidget(), "belvoWidget") // Used to listen for additional events
- [Optional] Add the following annotation where needed to suppress any warning:
@SuppressLint("JavascriptInterface")
✅ Done! You can now listen to additional events in your webview!
Updated 5 months ago